GUGRAPH* g = NULL;
FILE* fp = NULL;
/* create graph */
g = guCreateGraph();
/* open target file */
fp = fopen("target1.png","wb");
/* desc */
guSetDescription( g, "This is a description, which is broken up every
N+ characters. It can also be broken with a newline character. All kinda
stuff like dates, etc. could go here...");
guSetBackColor( g, 232, 255, 255 );
/* add a series */
guSetSeriesName( g, 25, "blah1");
guSetSeriesName( g, 27, "blah2");
guSetSeriesName( g, 28, "blah3");
guSetSeriesName( g, 29, "blah4");
guSetSeriesName( g, 30, "blah5");
guSetSeriesName( g, 31, "blah6");
//guSetSeriesName( g, 'S', "using ascii value of S as series id");
/* add more data */
guAddSeriesDataPoint( g, 27, 103, 2 );
guAddSeriesDataPoint( g, 27, 104, 0 );
guAddSeriesDataPoint( g, 27, 105, 9 );
guAddSeriesDataPoint( g, 27, 106, 9 );
guAddSeriesDataPoint( g, 27, 107, -1 );
guAddSeriesDataPoint( g, 27, 108, 9 );
/* add more data */
guAddSeriesDataPoint( g, 28, 103, 1 );
guAddSeriesDataPoint( g, 28, 104, 8 );
guAddSeriesDataPoint( g, 28, 105, 3 );
guAddSeriesDataPoint( g, 28, 106, -3 );
guAddSeriesDataPoint( g, 28, 107, -3 );
guAddSeriesDataPoint( g, 28, 108, .01 );
/* add more data */
guAddSeriesDataPoint( g, 40, 103, 5 );
guAddSeriesDataPoint( g, 40, 104, 8 );
guAddSeriesDataPoint( g, 40, 105, 3 );
guAddSeriesDataPoint( g, 40, 106, 3 );
guAddSeriesDataPoint( g, 40, 107, -4 );
guAddSeriesDataPoint( g, 40, 108, 0 );
/* add more data */
guAddSeriesDataPoint( g, 31, 103, 3 );
guAddSeriesDataPoint( g, 31, 104, 3 );
guAddSeriesDataPoint( g, 31, 105, 3 );
guAddSeriesDataPoint( g, 31, 106, 4 );
guAddSeriesDataPoint( g, 31, 107, 5 );
guAddSeriesDataPoint( g, 31, 108, 6 );
/* change bucket size */
guSetXBucketSize( g, .1 );
/* change bucket back */
guXBucketSizeAuto( g );
/* change image width */
guImageHeightDynamic( g );
guImageWidthDynamic( g );
/* grid */
guShowYGrid( g );
guShowXGrid( g );
/* descriptions */
guSetDescriptionCharsPerLine( g, 30 );
guAddYBucketDescription( g, 5, "bucket 5" );
/* set graph type */
guSetStyle( g, GU_STYLE_LINE_PLOT );
guSetYRange( g, -1,11 );
/* marker */
guSetMarker( g, 25, '+' );
guSetMarker( g, 27, 'X' );
guSetMarker( g, 28, '*' );
guSetMarker( g, 31, '.' );
guSetMarker( g, 40, '.' );
/* coordinates */
guShowPointCoordinates( g, 25 );
guShowPointCoordinates( g, 27 );
guShowPointCoordinates( g, 28 );
guShowPointCoordinates( g, 31 );
guShowPointCoordinates( g, 40 );
/* change marker colors */
guSetMarkerColor( g, 40, 0, 0, 0 );
guSetMarkerSize( g, 40, 6 );
guSetMarkerColor( g, 25, 0, 0, 0 );
/* Set log if desired */
//guXAxisLog( g );
//guXAxisLog( g );
/* change a few colors */
//guSetSeriesColor( g, 25, 255, 50, 50 );
//guSetSeriesColor( g, 27, 50, 255, 50 );
//guSetSeriesColor( g, 28, 50, 50, 255 );
/* open target file */
guWriteGraphPng(g,fp);
/* destroy graph and close file */
guDestroyGraph(g);
fclose(fp);
|
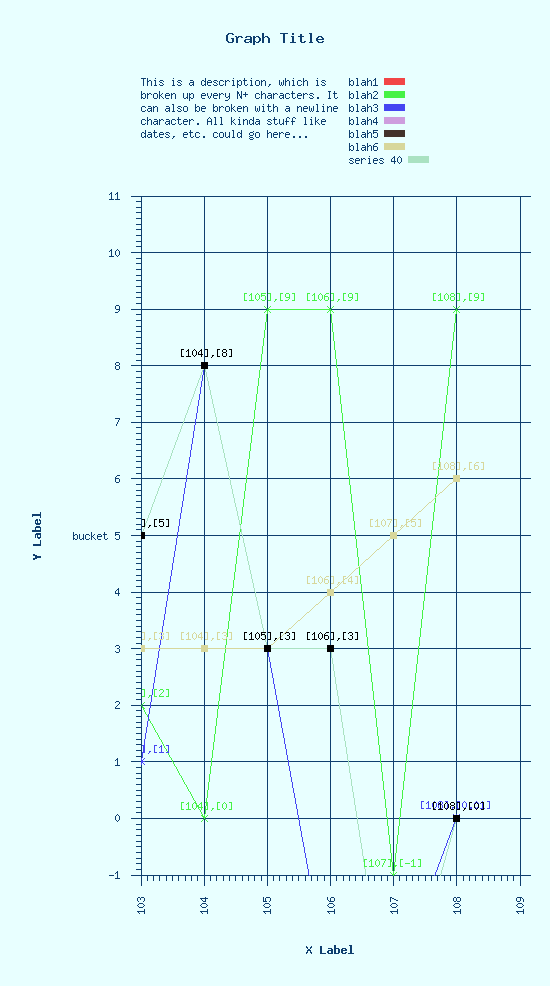 |